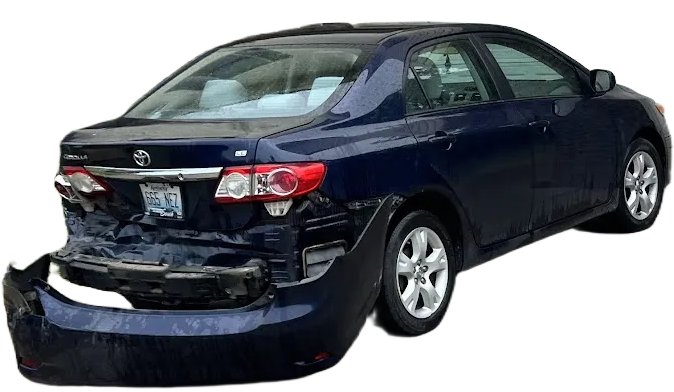
Night Mode
Added some new functionality and posts to the site. It's been a remarkably tough couple of months for me, but my spirit is undefeatable (regrettably.) I templated text, accent, and background colors to do dark mode but then decided to throw live color changing in there as well (the color picker up at the top.) I built in style persistance between pages using localStorage. I added a compiled list of all the songs from the "Songs for the Month" posts, and I switched away from using astro's ViewTransitions. Too much workaround code for simple functionality. Also O moved away from expandable grid stuff for the about me page and set up a modal instead.
I put together a powershell script to grab the songs from the markdown files as well. Got a couple of encoding errors this time around so I’ll try to fix it before I run it again.
# Define a function to extract song information from a file
function Extract-SongsFromFile {
param (
[string]$filePath
)
Write-Output "Reading file: $filePath"
$content = Get-Content -Path $filePath -Raw
# Generalized regular expression to match the song information
$pattern = '<iframe.*?src="(.*?)".*?</iframe>\s*<h2>\s*(.*?)\s*</h2>\s*<h3>\s*by\s*(.*?)\s*</h3>'
Write-Output "Applying regex pattern to extract song information..."
$matches = [regex]::Matches($content, $pattern)
if ($matches.Count -eq 0) {
Write-Output "No matches found in file: $filePath"
}
$songs = @()
foreach ($match in $matches) {
$song = @{
youtube_url = $match.Groups[1].Value.Trim()
title = $match.Groups[2].Value.Trim()
artist = $match.Groups[3].Value.Trim()
}
Write-Output "Extracted song: Title='$($song.title)', Artist='$($song.artist)', YouTube URL='$($song.youtube_url)'"
$songs += $song
}
return $songs
}
# Main script
$directory = ".\src\content\blog\"
Write-Output "Searching for files in directory: $directory"
$songFiles = Get-ChildItem -Path $directory -Filter 'songs-for-the-month*.md'
Write-Output "Found files:"
$songFiles | ForEach-Object {
Write-Output $_.FullName
}
$allSongs = @()
foreach ($file in $songFiles) {
$songs = Extract-SongsFromFile -filePath $file.FullName
$allSongs += $songs
}
Write-Output "All extracted songs:"
$allSongs | ForEach-Object {
Write-Output "Title: $($_.title)"
Write-Output "Artist: $($_.artist)"
Write-Output "YouTube URL: $($_.youtube_url)"
Write-Output ""
}
# Convert the results to JSON
$jsonOutput = $allSongs | ConvertTo-Json -Depth 3
Write-Output "JSON Output:"
Write-Output $jsonOutput
# Save the JSON output to a file
$jsonOutput | Out-File -FilePath ".\extracted_songs.json"
Write-Output "JSON output saved to extracted_songs.json"